### Ako zvládnuť webinár
* pomenuj svojho avatara
* napr. dvojkliknutím na svoje meno vpravo hore
* nevysielaj obraz, pokiaľ nemusíš
* ušetríš linku ostatným ;)
* vypni mikrofón, keď nehovoríš
* ušetríš linku ostatným ;)
* minimalizuješ vzruchy
* pýtaj sa, ak máš nejasnosti
* stlač medzerník a drž ho, kým rozprávaš (ako na vysielačke) alebo použi chat
## Tvorba webových stránok v jazyku Python
mirek@cnl.sk / [**Namakaný deň - Webináre**](http://www.namakanyden.sk/webinare/)
Newsletter
[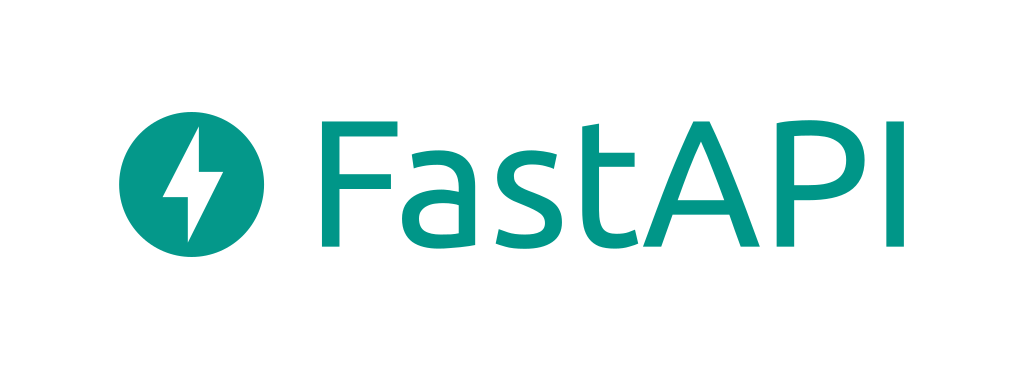](https://fastapi.tiangolo.com)
FastAPI framework, high performance, easy to learn, fast to code, ready for production
## Features
[Fast](https://www.techempower.com/benchmarks/#section=test&runid=7464e520-0dc2-473d-bd34-dbdfd7e85911&hw=ph&test=query&l=z8kflr-v&a=2),
Fast to code, Fewer bugs, Intuitive, Easy, Short, Robust, Standards-based
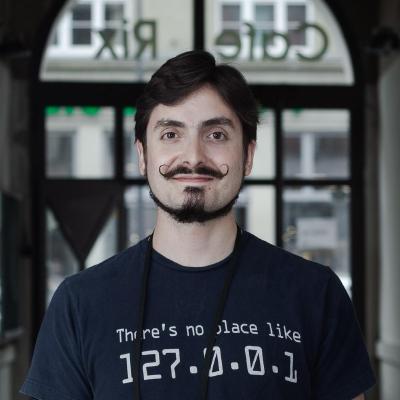]
## [Sebastian Ramirez](https://github.com/tiangolo)