## Microservices with FastAPI
[Mirek Biňas](https://bletvaska.github.io)
/ [**Namakaný webinár**](http://www.namakanyden.sk/)
## The Traditional Monolith Approach
## Microservices
> ...a collection of **loosely coupled specialized services** that **work in unison** to provide a comprehensive service.
> -- [J. Buelta: Hands-On Docker for Microservices with Python (2019)](https://www.amazon.com/Hands-Docker-Microservices-Python-microservices/dp/1838823816)
> ...is an approach to developing a single application as a **suite of small services**, each **running in its own process** and communicating with lightweight mechanisms, often an HTTP resource API. These services are **built around business capabilities** and **independently deployable** by fully automated deployment machinery. There is a **bare minimum of centralized management** of these services, which may be written in different programming languages and use different data storage technologies.
> -- [J. Lewis and M. Fowler (2014)](https://martinfowler.com/articles/microservices.html)
## REST API
A **REST API** (also known as RESTful API) is an **application programming interface** (API or web API) that conforms to the constraints of **REST architectural style** and allows for interaction with RESTful web services.
notes:
* https://www.redhat.com/en/topics/api/what-is-a-rest-api
* REST stands for representational state transfer and was created by computer scientist Roy Fielding.
[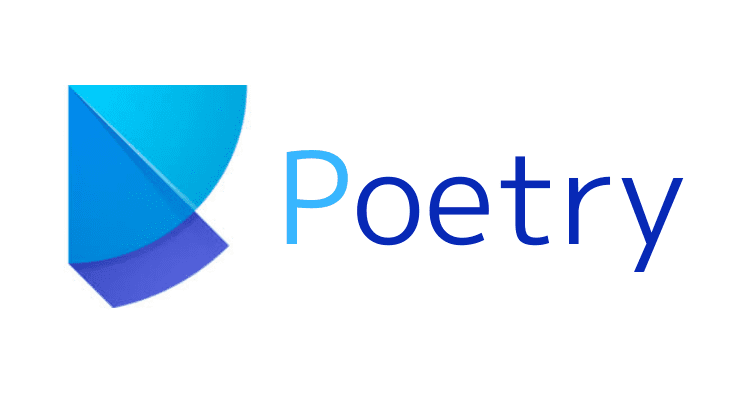](https://python-poetry.org/)
## [HTTP Status Codes](https://httpstatuses.com/)
[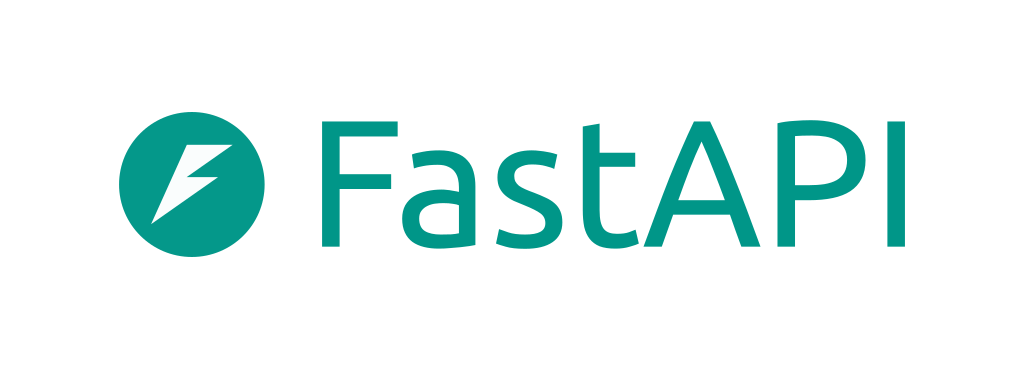](https://fastapi.tiangolo.com/)
FastAPI framework, high performance, easy to learn, fast to code, ready for production
## Features
[Fast](https://www.techempower.com/benchmarks/#section=data-r20&hw=ph&test=query&l=v2qiv3-db&a=2),
Fast to code, Fewer bugs, Intuitive, Easy, Short, Robust, Standards-based
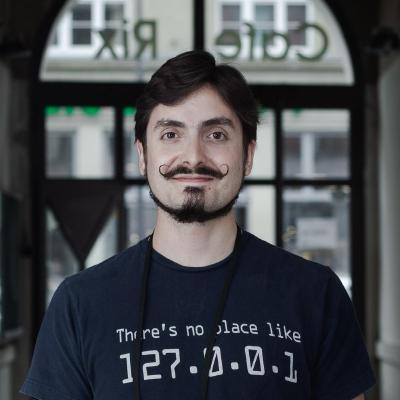
## [Sebastian Ramirez](https://github.com/tiangolo)